CupertinoButton
An iOS-style button.
Examples
Basic Example
- Python
import flet as ft
def main(page: ft.Page):
page.add(
ft.CupertinoButton(
content=ft.Text("Normal CupertinoButton", color=ft.cupertino_colors.DESTRUCTIVE_RED),
opacity_on_click=0.3,
on_click=lambda e: print("Normal CupertinoButton clicked!"),
),
ft.CupertinoButton(
content=ft.Text("Filled CupertinoButton", color=ft.colors.YELLOW),
bgcolor=ft.colors.PRIMARY,
alignment=ft.alignment.top_left,
border_radius=ft.border_radius.all(15),
opacity_on_click=0.5,
on_click=lambda e: print("Filled CupertinoButton clicked!"),
),
ft.ElevatedButton(
adaptive=True, # a CupertinoButton will be rendered when running on apple-platform
bgcolor=ft.cupertino_colors.SYSTEM_TEAL,
content=ft.Row(
[
ft.Icon(name=ft.icons.FAVORITE, color="pink"),
ft.Text("ElevatedButton+adaptive"),
],
tight=True,
),
),
)
ft.app(main)
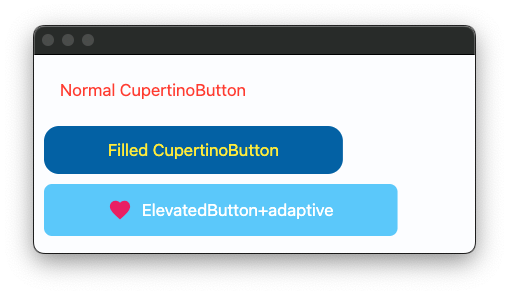
Properties
bgcolor
Button's background color.
color
Button's text color.
disabled_bgcolor
The background color of the button when it is disabled.
disabled_color
disabled_color
The background color of the button when it is disabled.
Deprecated in v0.24.0 and will be removed in v0.27.0. Use disabled_bgcolor
instead.
content
A Control representing custom button content.
icon
Icon shown in the button.
icon_color
Icon color.
min_size
The minimum size of the button.
Defaults to 44.0
.
opacity_on_click
Defines the opacity of the button when it is clicked. When not pressed, the button has an opacity of 1.0
.
Defaults to 0.4
.
padding
The amount of space to surround the content
control inside the bounds of the button.
text
The text displayed on a button.
tooltip
The text displayed when hovering the mouse over the button.
url
The URL to open when the button is clicked. If registered, on_click
event is fired after that.
url_target
Where to open URL in the web mode.
Value is of type UrlTarget
and defaults to UrlTarget.BLANK
.
Events
on_click
Fires when a user clicks the button.